# CSS 预处理器
JsView的CSS预处理器是从Vue参考而来,主要支持Sass、Less、Stylus等。
# 1. 预备知识
如果你想了解CSS预处理器的基础知识,可以通过自己的方式或下方的链接了解其中一个或几个相关知识:
# 2. 安装
cd [jsview-project]
npm install --save-dev sass sass-loader@10.2.1
2
cd [jsview-project]
npm install --save-dev less less-loader@7.3.0
2
cd [jsview-project]
npm install --save-dev stylus stylus-loader@4.3.3
2
然后你就可以在 *.vue 文件中这样来使用:
<style scoped lang="scss">
</style>
2
<style scoped lang="less">
</style>
2
<style scoped lang="stylus">
</style>
2
注意
为了加速CSS的渲染,<style scoped> 中的 scoped 关键字是必须的,否则将不处理CSS的继承功能。
# 3. 常用功能
注意
JsView只支持CSS .class 形式的类选择器,所以在预处理器中也只支持类选择器。 关于CSS在JsView中的使用限制,请参照 JS开发功能限制说明-CSS样式
# **. 功能一览
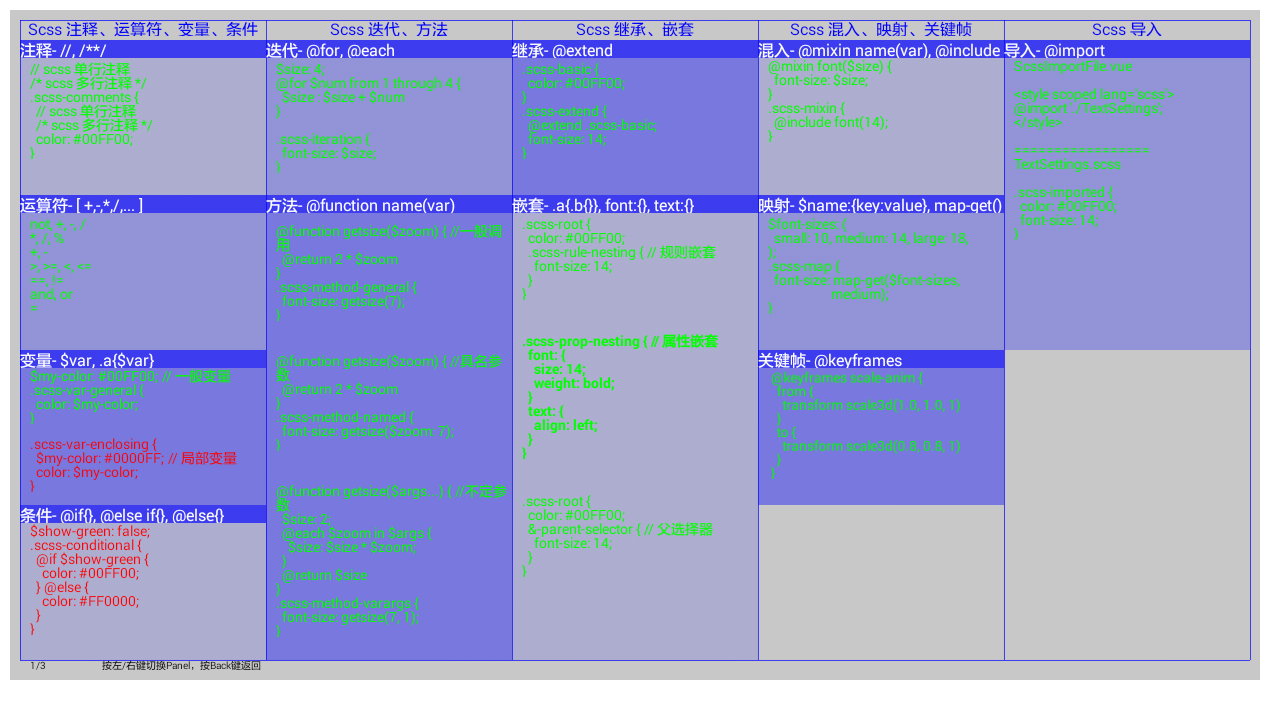
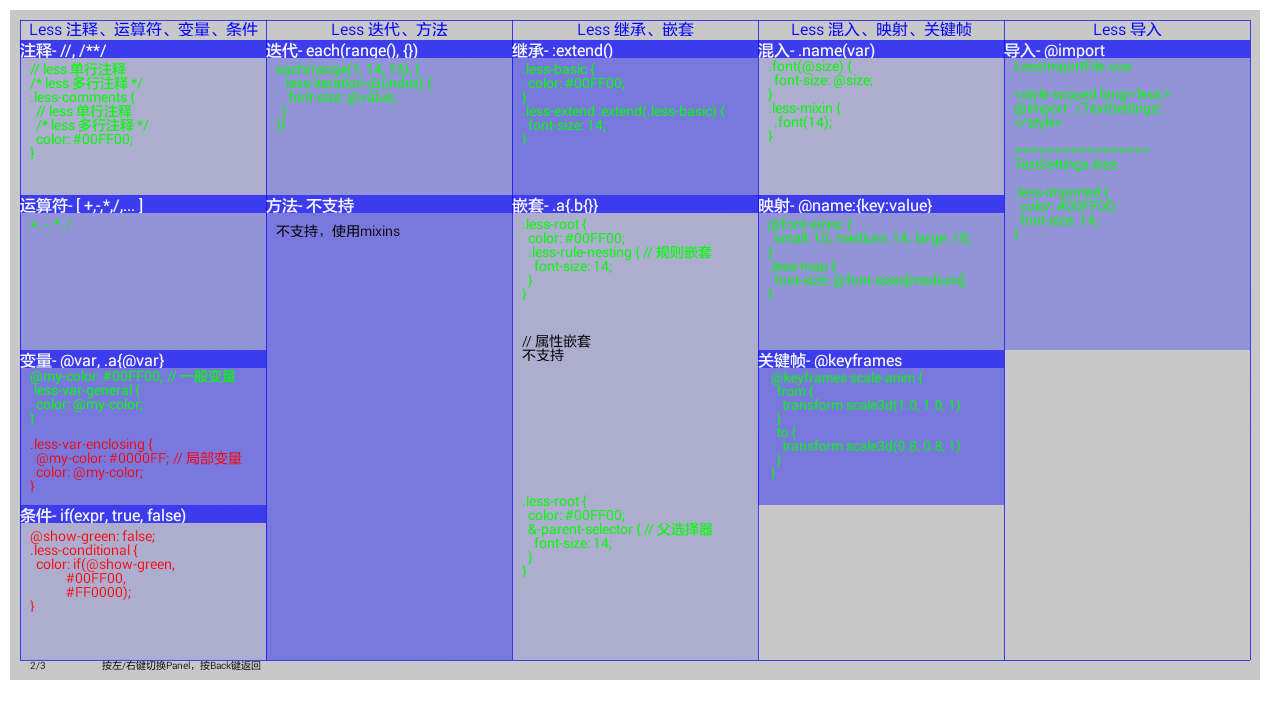
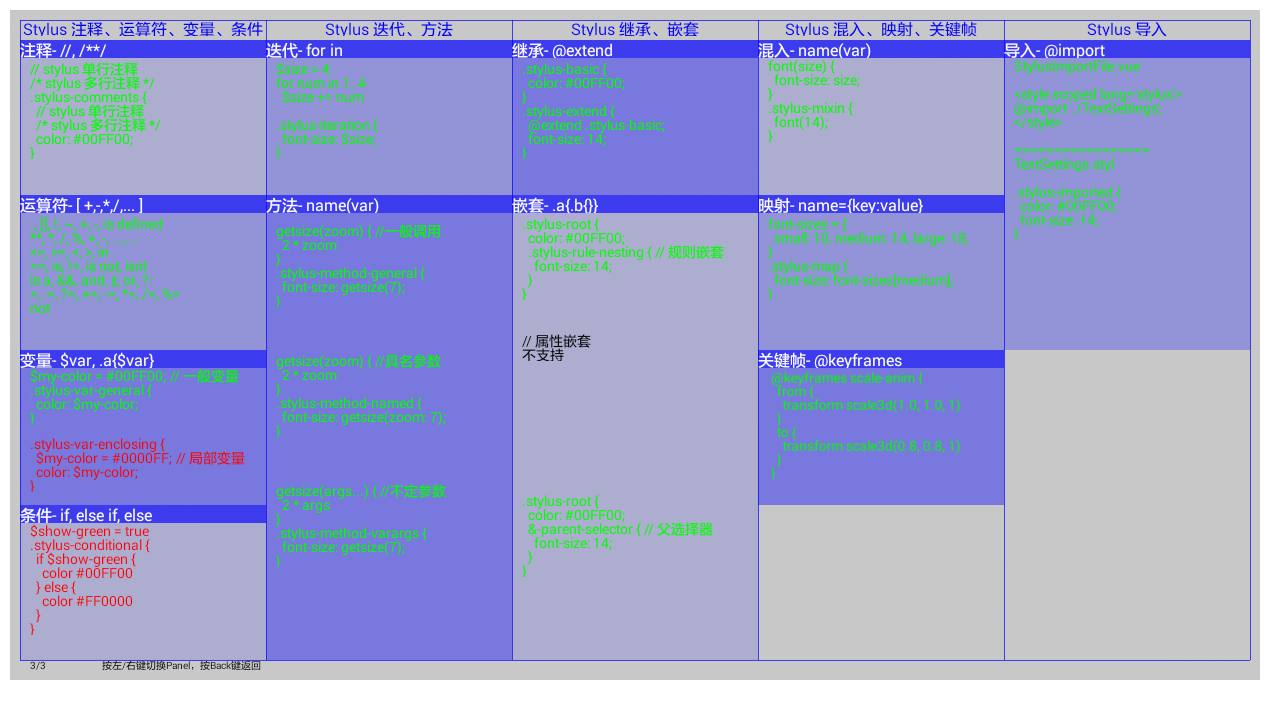
# 01. 注释(Comments)
参考文档: https://sass-lang.com/documentation/syntax/comments (opens new window)
// scss 单行注释
/* scss
* 多行注释 */
.scss-comments {
// scss 单行注释
/* scss
* 多行注释 */
}
2
3
4
5
6
7
8
参考文档: https://lesscss.org/#comments (opens new window)
// less 单行注释
/* less
* 多行注释 */
.less-comments {
// less 单行注释
/* less
* 多行注释 */
}
2
3
4
5
6
7
8
参考文档: https://stylus-lang.com/docs/comments.html (opens new window)
// stylus 单行注释
/* stylus
* 多行注释 */
.stylus-comments {
// stylus 单行注释
/* stylus
* 多行注释 */
}
2
3
4
5
6
7
8
# 02. 运算符(Operators)
参考文档: https://sass-lang.com/documentation/operators (opens new window)
not, +, -, /
*, /, %
+, -
>, >=, <, <=
==, !=
and, or
=
2
3
4
5
6
7
参考文档: https://lesscss.org/#operations (opens new window)
+, -, *, /
参考文档: https://stylus-lang.com/docs/operators.html (opens new window)
.
[]
!, ~, +, -
is defined
**, *, /, %
+, -
..., ..
<=, >=, <, >
in
==, is, !=, is not, isnt
is a
&&, and, ||, or
?:
=, :=, ?=, +=, -=, *=, /=, %=
not
2
3
4
5
6
7
8
9
10
11
12
13
14
15
# 03. 变量(Variables)
参考文档: https://sass-lang.com/documentation/variables (opens new window)
$my-color: #00FF00; // 一般变量
.scss-var-general {
color: $my-color;
}
.scss-var-enclosing {
$my-color: #0000FF; // 局部变量
color: $my-color;
}
2
3
4
5
6
7
8
9
参考文档: https://lesscss.org/#variables (opens new window)
@my-color: #00FF00; // 一般变量
.less-var-general {
color: @my-color;
}
.less-var-enclosing {
@my-color: #0000FF; // 局部变量
color: @my-color;
}
2
3
4
5
6
7
8
9
参考文档: https://stylus-lang.com/docs/variables.html (opens new window)
$my-color = #00FF00; // 一般变量
.stylus-var-general {
color: $my-color;
}
.stylus-var-enclosing {
$my-color = #0000FF; // 局部变量
color: $my-color;
}
2
3
4
5
6
7
8
9
# 04. 条件(Conditionals)
参考文档: https://sass-lang.com/documentation/at-rules/control/if#else (opens new window)
$show-green: false;
.scss-conditional {
@if $show-green {
color: #00FF00;
} @else {
color: #FF0000;
}
}
2
3
4
5
6
7
8
参考文档: https://lesscss.org/functions/#logical-functions-if (opens new window)
@show-green: false;
.less-conditional {
color: if(@show-green,
#00FF00,
#FF0000);
}
2
3
4
5
6
参考文档: https://stylus-lang.com/docs/conditionals.html (opens new window)
$show-green = false
.stylus-conditional {
if $show-green {
color: #00FF00;
} else {
color: #FF0000;
}
}
2
3
4
5
6
7
8
# 05. 迭代(Iteration)
参考文档: https://sass-lang.com/documentation/at-rules/control/for (opens new window)
$size: 4;
@for $num from 1 through 4 {
$size : $size + $num
}
.scss-iteration {
font-size: $size;
}
2
3
4
5
6
7
8
参考文档: https://lesscss.org/functions/#list-functions-each (opens new window)
each(range(1, 14, 13), {
.less-iteration-@{index} {
font-size: @value;
}
})
2
3
4
5
参考文档: https://stylus-lang.com/docs/iteration.html (opens new window)
$size = 4
for num in 1..4
$size += num
.stylus-iteration {
font-size: $size;
}
2
3
4
5
6
7
# 06. 方法(Methods)
参考文档: https://sass-lang.com/documentation/at-rules/function#arguments (opens new window)
@function getsize($zoom) { //一般调用
@return 2 * $zoom
}
.scss-method-general {
font-size: getsize(7);
}
@function getsize($zoom) { //具名参数
@return 2 * $zoom
}
.scss-method-named {
font-size: getsize($zoom: 7);
}
@function getsize($args...) { //不定参数
$size: 2;
@each $zoom in $args {
$size: $size * $zoom;
}
@return $size
}
.scss-method-varargs {
font-size: getsize(7, 1);
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
参考文档: 无
不支持,使用mixins
参考文档: https://stylus-lang.com/docs/functions.html (opens new window)
getsize(zoom) { //一般调用
2 * zoom
}
.stylus-method-general {
font-size: getsize(7);
}
getsize(zoom) { //具名参数
2 * zoom
}
.stylus-method-named {
font-size: getsize(zoom: 7);
}
getsize(args...) { //不定参数
2 * args
}
.stylus-method-varargs {
font-size: getsize(7);
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
- 内置函数(Buildin-Functions)
# 07. 继承(Extends)
参考文档: https://sass-lang.com/documentation/at-rules/extend (opens new window)
.scss-basic {
color: #00FF00;
}
.scss-extend {
@extend .scss-basic;
font-size: 14;
}
2
3
4
5
6
7
8
参考文档: https://lesscss.org/features/#extend-feature (opens new window)
.less-basic {
color: #00FF00;
}
.less-extend :extend(.less-basic) {
font-size: 14;
}
2
3
4
5
6
7
参考文档: https://stylus-lang.com/docs/extend.html (opens new window)
.stylus-basic {
color: #00FF00;
}
.stylus-extend {
@extend .stylus-basic
font-size: 14;
}
2
3
4
5
6
7
8
# 08. 嵌套(Nesting)
参考文档: https://sass-lang.com/documentation/style-rules#nesting (opens new window)
.scss-root {
color: #00FF00;
.scss-rule-nesting { // 规则嵌套
font-size: 14;
}
}
.scss-prop-nesting { // 属性嵌套
font: {
size: 14;
weight: bold;
}
text: {
align: left;
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
参考文档: https://lesscss.org/#nesting (opens new window)
less-root {
color: #00FF00;
.less-rule-nesting { // 规则嵌套
font-size: 14;
}
}
// 属性嵌套
不支持
2
3
4
5
6
7
8
9
参考文档: 无
.stylus-root {
color: #00FF00;
.stylus-rule-nesting { // 规则嵌套
font-size: 14;
}
}
// 属性嵌套
不支持
2
3
4
5
6
7
8
9
- 父选择器
参考文档: https://sass-lang.com/documentation/style-rules/parent-selector (opens new window)
.scss-root {
color: #00FF00;
&-parent-selector { // 父选择器
font-size: 14;
}
}
2
3
4
5
6
参考文档: https://lesscss.org/features/#parent-selectors-feature (opens new window)
.less-root {
color: #00FF00;
&-parent-selector { // 父选择器
font-size: 14;
}
}
2
3
4
5
6
参考文档: https://stylus-lang.com/docs/mixins.html#parent-references (opens new window)
.stylus-root {
color: #00FF00;
&-parent-selector { // 父选择器
font-size: 14;
}
}
2
3
4
5
6
# 09. 混入(Mixins)
参考文档: https://sass-lang.com/documentation/at-rules/mixin (opens new window)
@mixin font($size) {
font-size: $size;
}
.scss-mixin {
@include font(14);
}
2
3
4
5
6
7
参考文档: https://lesscss.org/#mixins (opens new window)
.font(@size) {
font-size: @size;
}
.less-mixin {
.font(14);
}
2
3
4
5
6
7
参考文档: https://stylus-lang.com/docs/mixins.html (opens new window)
font(size) {
font-size: size;
}
.stylus-mixin {
font(14);
}
2
3
4
5
6
7
# 10. 映射(Maps)
参考文档: https://sass-lang.com/documentation/values/maps (opens new window)
$font-sizes: (
small: 10, medium: 14, large: 18,
);
.scss-map {
font-size: map-get($font-sizes,
medium);
}
2
3
4
5
6
7
8
参考文档: https://lesscss.org/#maps (opens new window)
@font-sizes: {
small: 10; medium: 14; large: 18;
}
.less-map {
font-size: @font-sizes[medium];
}
2
3
4
5
6
7
参考文档: https://stylus-lang.com/docs/hashes.html (opens new window)
font-sizes = {
small: 10, medium: 14, large: 18,
}
.stylus-map {
font-size: font-sizes[medium];
}
2
3
4
5
6
7
# 11. 关键帧(Keyframes)
参考文档: https://sass-lang.com/documentation/at-rules/css#keyframes (opens new window)
@keyframes scale-anim {
from {
transform: scale3d(1.0, 1.0, 1);
}
to {
transform: scale3d(0.8, 0.8, 1);
}
}
2
3
4
5
6
7
8
参考文档: 无
@keyframes scale-anim {
from {
transform: scale3d(1.0, 1.0, 1);
}
to {
transform: scale3d(0.8, 0.8, 1);
}
}
2
3
4
5
6
7
8
参考文档: https://stylus-lang.com/docs/keyframes.html (opens new window)
@keyframes scale-anim {
from {
transform: scale3d(1.0, 1.0, 1);
}
to {
transform: scale3d(0.8, 0.8, 1);
}
}
2
3
4
5
6
7
8
# 12. 导入(Importing)
参考文档: https://sass-lang.com/documentation/at-rules/import (opens new window)
ScssImportFile.vue
<style scoped lang='scss'>
@import './TextSettings';
</style>
2
3
TextSettings.scss
.scss-imported {
color: #00FF00;
font-size: 14;
}
2
3
4
参考文档: https://lesscss.org/#importing (opens new window)
LessImportFile.vue
<style scoped lang='less'>
@import './TextSettings';
</style>
2
3
TextSettings.less
.less-imported {
color: #00FF00;
font-size: 14;
}
2
3
4
参考文档: https://stylus-lang.com/docs/import.html (opens new window)
StylusImportFile.vue
<style scoped lang='stylus'>
@import './TextSettings';
</style>
2
3
TextSettings.styl
.stylus-imported {
color: #00FF00;
font-size: 14;
}
2
3
4
# 13. 其他(Others)
- 对于其他的预处理器功能,JsView并没有做兼容性测试。如果再使用过程中发生问题,请提交到这里 (opens new window)。